ESP32의 최대 장점인 와이파이를 연결하여 아주 간단한 웹서버를 만들겠습니다.
아래의 소스코드를 ESP32 모듈에 넣고 동작 시킵니다.
시리얼 모니터에 표시되는 IP 주소를 웹브라우저(저는 크롬 사용)의 주소창에 입력하면
프로그래머에게 너무나 친숙한 'Hello world'가 표시 됩니다.
#include <WiFi.h>
#include <AsyncTCP.h>
#include <ESPAsyncWebServer.h>
AsyncWebServer server(80);
const char* ssid = "YOUR_SSID";
const char* password = "YOUR_PASSWORD";
// 페이지를 찾을 수 없을 때
void notFound(AsyncWebServerRequest *request) {
request->send(404, "text/plain", "Not found");
}
void setup() {
Serial.begin(115200);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
// 와이파이가 연결될 때까지 기다린다.
if (WiFi.waitForConnectResult() != WL_CONNECTED) {
Serial.printf("WiFi Failed!\n");
return;
}
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
request->send(200, "text/plain", "Hello world");
});
server.onNotFound(notFound);
server.begin(); // 서버 시작
}
void loop() {
}
실행된 화면
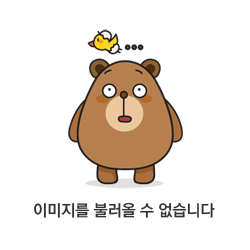
먼저, 'AsyncTCP' 와 'ESPAsyncWebServer' 라이브러리를 설치 하여 줍니다.
라이브러리 추가 방법
https://jooduino.tistory.com/4
아두이노 라이브러리 추가 방법
아두이노 IDE에 라이브러리 등록 방법 입니다. 라이브러리 등록 방법은 두 가지 입니다. 1. 라이브러리 매니저를 이용하는 방법. 2. 다운로드 받은 zip 파일 추가. 라이브러리 매니저를 이용하는 방
jooduino.tistory.com
#include <AsyncTCP.h>
#include <ESPAsyncWebServer.h>
현재 사용하는 공유기의 'SSID' 와 '패스워드'를 입력합니다.
const char* ssid = "YOUR_SSID";
const char* password = "YOUR_PASSWORD";
'Hello world' 글자를 원하는 다른 글자로 바꾸어 보세요.
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
request->send(200, "text/plain", "안녕하세요?");
});
한글로 바꾸어 보면 브라우저에 깨진 글자가 출력 됩니다.
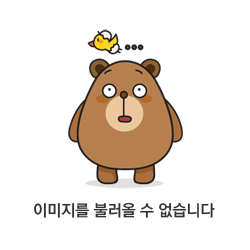
한글로 출력하고 싶으시면 html 형식으로 바꾸시면 됩니다.
아래의 코드를 추가 하고
const char index_html[] PROGMEM = R"==(
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>ESP32 테스트 페이지</title>
</head>
<body>
<h1>안녕하세요?</h1>
</body>
</html>
)==";
코드를 이렇게 수정합니다.
request->send(200, "text/html", index_html);
실행된 화면
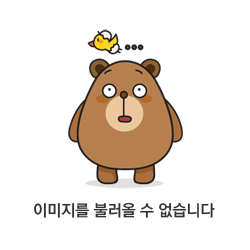
전체 소스코드 입니다.
#include <WiFi.h>
#include <AsyncTCP.h>
#include <ESPAsyncWebServer.h>
AsyncWebServer server(80);
const char* ssid = "YOUR_SSID";
const char* password = "YOUR_PASSWORD";
const char index_html[] PROGMEM = R"==(
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>ESP32 테스트 페이지</title>
</head>
<body>
<h1>안녕하세요?</h1>
</body>
</html>
)==";
// 페이지를 찾을 수 없을 때
void notFound(AsyncWebServerRequest *request) {
request->send(404, "text/plain", "Not found");
}
void setup() {
Serial.begin(115200);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
// 와이파이가 연결될 때까지 기다린다.
if (WiFi.waitForConnectResult() != WL_CONNECTED) {
Serial.printf("WiFi Failed!\n");
return;
}
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
request->send(200, "text/html", index_html);
});
server.onNotFound(notFound);
server.begin(); // 서버 시작
}
void loop() {
}
이제는 한글이 잘 출력 되는걸 볼 수 있습니다.
다음 강좌에서는 HTML을 이용하여 버튼을 만들어서 버튼을 눌러 LED를 ON/OFF 을 해보겠습니다.
'ESP32 > 펌웨어' 카테고리의 다른 글
ESP32 OTA를 이용한 펌웨어 업데이트 (2) | 2023.10.03 |
---|---|
ESP32 웹서버 만들기 - 입력 받기 (0) | 2023.10.03 |
아두이노 버튼 라이브러리 (0) | 2023.10.03 |
ESP32 웹서버 만들기 - CSS를 이용하여 버튼 예쁘게 (0) | 2023.09.26 |
ESP32 웹서버 만들기 - 버튼 추가 (0) | 2023.09.24 |